Picture this: Your team has just launched an impressive new web application. Users are flocking to it. Then comes the news no developer wants to hear—someone has compromised your authentication system and accessed sensitive user data.
This scenario plays out far too often in today’s digital landscape. And frequently, it stems from one critical architectural decision: where you handle your authentication.
In this comprehensive guide, we’ll explore why managing authentication on your backend (using the “Backend for Frontend” or BFF pattern) offers substantially better security than handling sensitive authentication logic in your frontend JavaScript. We’ll break down the security implications, examine real-world examples, and provide practical implementation guidance—all in straightforward language that both technical and non-technical readers can appreciate.
The Critical Authentication Decision: Frontend vs. Backend
Before diving into the security implications, let’s clarify the two main approaches to authentication in modern web applications:
The Frontend-Only Approach
User → Frontend App → Auth Server → Protected Resources
(stores tokens)
With this approach:
- Your frontend JavaScript handles the entire authentication flow
- Access tokens, ID tokens, and refresh tokens live in the browser
- Your frontend code refreshes tokens when they expire
- Your frontend attaches tokens to API requests
This approach gained popularity because it seems simpler initially—no need for a dedicated backend, right? Many Single Page Applications (SPAs) built with React, Angular, or Vue have historically used this pattern because it aligns with the “serverless” philosophy.
The Backend-for-Frontend (BFF) Approach
User → Frontend App → Backend → Auth Server → Protected Resources
(stores tokens)
In this model:
- Your frontend initiates login but only receives a session cookie
- A backend server exchanges the auth code for tokens and manages them
- Refresh tokens never touch the browser—they stay safely on your server
- Your backend attaches the right tokens when proxying API requests
This approach is what major tech companies like Google, Facebook, and Microsoft use for their web applications. But why exactly do they choose this architecture?
Understanding the OAuth 2.0 and OpenID Connect Context
To fully appreciate the security implications, we need to understand how modern authentication protocols work. Most applications today use OAuth 2.0 for authorization and OpenID Connect (OIDC) for authentication.
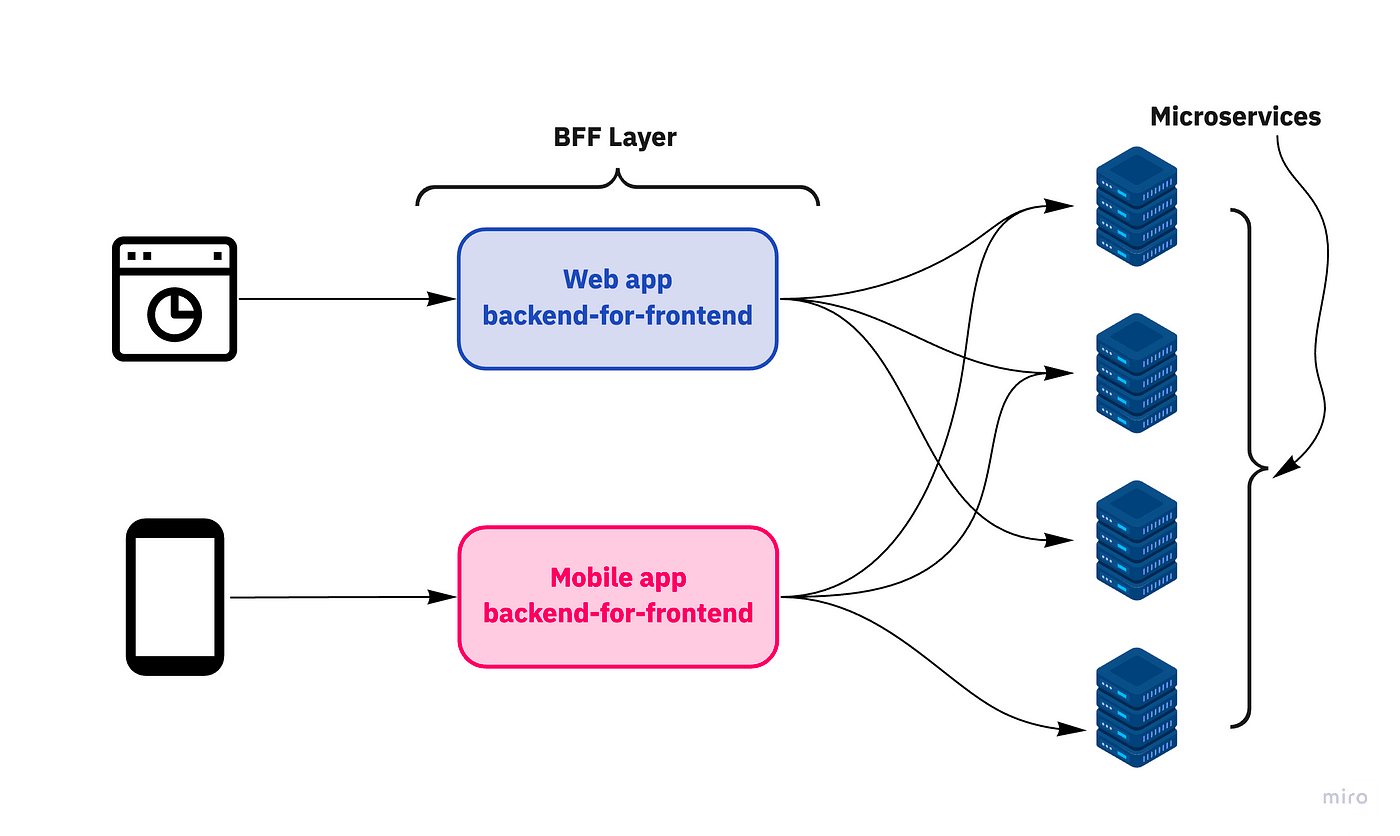
These protocols involve several types of tokens:
- Access tokens: Short-lived credentials that grant access to protected resources
- ID tokens: Contain user identity information (in OIDC flows)
- Refresh tokens: Long-lived tokens that can obtain new access tokens
The OAuth 2.0 specification classifies client applications as either:
- Confidential clients: Applications that can securely store client secrets (like server-side applications)
- Public clients: Applications that cannot securely store secrets (like browser-based or mobile applications)
This distinction is crucial because it acknowledges an important truth: applications running in user browsers operate in an inherently insecure environment.
The Security Case for Backend Authentication
1. Your Tokens Are Exposed to Browser Threats
Browser-based storage options all have security vulnerabilities:
- localStorage/sessionStorage: Completely vulnerable to cross-site scripting (XSS) attacks. If an attacker can inject JavaScript into your page—even through a third-party library—they can steal tokens.
- Cookies: Better, but still vulnerable to Cross-Site Request Forgery (CSRF) attacks unless perfectly configured with proper flags.
Auth0, a leading identity platform, explains: “The Backend For Frontend pattern for authentication emerged to mitigate any risk that may occur from negotiating and handling access tokens from public clients running in a browser.”
The IETF’s “OAuth 2.0 for Browser-Based Applications” draft explicitly states that previous recommendations about storing tokens in browser storage “are often no longer recommended” due to evolving security threats.
Consider this sobering statistic: According to a study by WhiteHat Security, Cross-Site Scripting remains among the top three most common web application vulnerabilities, affecting 11% of applications. With frontend authentication, an XSS vulnerability anywhere in your application or its dependencies can lead directly to compromised authentication tokens.
2. Refresh Tokens Are High-Value Targets
Refresh tokens are particularly valuable to attackers because they:
- Have long lifespans (days, weeks, or even months)
- Can obtain new access tokens without user login
- Provide persistent access to user accounts
Imagine giving someone a key that not only opens your house but can also make copies of itself. That’s essentially what a compromised refresh token represents.
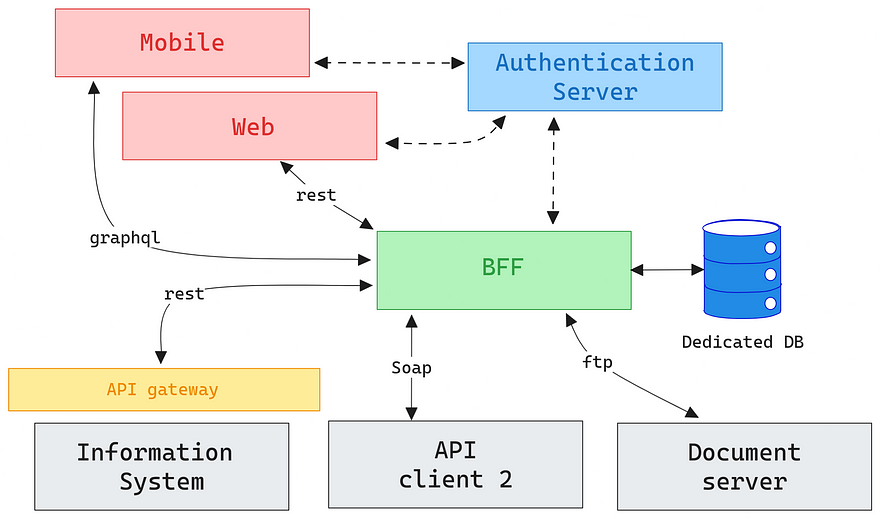
The OAuth 2.0 Security Best Current Practice document explicitly recommends against storing refresh tokens in browser applications:
“Refresh tokens should not be issued to public clients… unless additional measures… are taken.”
In a recent security incident analyzed by OWASP, attackers specifically targeted refresh tokens stored in browser localStorage, gaining prolonged access to victim accounts even after passwords were changed. This type of attack becomes impossible when refresh tokens never reach the browser.
3. The Browser Is an Inherently Insecure Environment
Browsers were designed primarily for content consumption, not secure credential storage. They operate in an environment where:
- Third-party JavaScript can be executed (analytics, plugins, etc.)
- Extensions can potentially access page content
- The security boundaries between sites can be compromised through various attack vectors
- Local storage can be inspected using simple developer tools
Baeldung, a respected technical resource, points out: “When inspecting with a debugging tool, we won’t find any Bearer tokens on any of the major websites known for using OAuth2 (Google, Facebook, Github, or LinkedIn). This is because according to security experts, we should not configure applications running on user devices as ‘public’ OAuth2 clients, even with PKCE.”
4. Server-Side Token Validation Is More Reliable
When tokens are validated server-side:
- The validation code isn’t exposed to end users
- Tokens can be immediately invalidated if suspicious activity is detected
- User permissions can be double-checked on each request
- Additional security checks can be implemented without exposing the logic
Frontend validation alone means trusting client-side code that could potentially be tampered with. As a fundamental security principle, we should never trust the client.
According to a report by Verizon’s Data Breach Investigations, 43% of data breaches involve web applications. Proper token validation is a critical defense mechanism, and it’s more robustly implemented on the server.
5. Better Security Controls and Monitoring
When authentication happens on your backend:
- You can implement more sophisticated validation
- You can revoke access immediately if suspicious activity is detected
- You have a central place to log authentication events for security monitoring
- You can enforce consistent security policies across all clients
This centralized control is invaluable for security incident response. If you detect suspicious activity, you can immediately revoke all tokens for affected users—something that’s nearly impossible with frontend authentication, where tokens could be stored across multiple devices and browsers.
The BFF Pattern: Practical Benefits Beyond Security
Beyond the core security advantages, the BFF pattern offers several additional technical benefits:
1. Token Lifecycle Management
Your backend can handle the entire token lifecycle—issuance, validation, refresh, and revocation—without exposing these operations to the client. This creates a more robust system where tokens can be proactively refreshed before they expire, preventing user disruption.
According to Auth0: “The BFF interacts with the authorization server as a confidential OAuth client and manages OAuth access and refresh tokens in the context of a cookie-based session, avoiding the direct exposure of any tokens to the JavaScript application.”
This approach is particularly valuable for handling edge cases like network reconnections, temporary service unavailability, or clock skew issues between servers.
2. Comprehensive Session Management
The backend can maintain robust session state that persists even when the frontend application reloads or crashes. This eliminates one of the most common user experience issues with frontend-only auth: having to re-authenticate after a page refresh.
As Zuplo’s blog notes: “The big change with BFF authentication is that you no longer need to juggle all of this authentication logic on the client – you authenticate the user, store some session state on the server, and use good old browser cookies to handle API authentication.”
3. Simplified User Experience
With proper implementation, users get a seamless experience because:
- They receive a secure, HttpOnly session cookie after login
- This cookie is automatically included with requests
- Your backend validates the session and attaches the right token
- Token refreshing happens automatically on the server
“BFF Authentication offers a way to simplify your auth code while enhancing security, and continuing to provide your end users with a nice login experience,” notes the Zuplo blog.
A study by the Baymard Institute found that complex authentication processes contribute significantly to checkout abandonment rates, which average around 70%. By hiding the complexity of token management on the server, you provide users with a simpler, more reliable experience.
4. Reduced Development Complexity
When authentication logic is centralized on the backend:
- Frontend teams can focus on user experience rather than security details
- Authentication code is written once, not duplicated across platforms
- Testing and validation are simpler with fewer moving parts
- Regulatory compliance is easier to demonstrate and maintain
The GitHub repository for an OIDC BFF implementation explains: “The security benefits of the OIDC BFF are: OIDC tokens and client secrets are kept at the backend, which should be more secure than the browser. The security session between browser and BFF is a ‘HTTP-only’ cookie, i.e. this is not available to potential malicious Javascript.”
“But It’s More Complex!” — Addressing the Complexity Argument
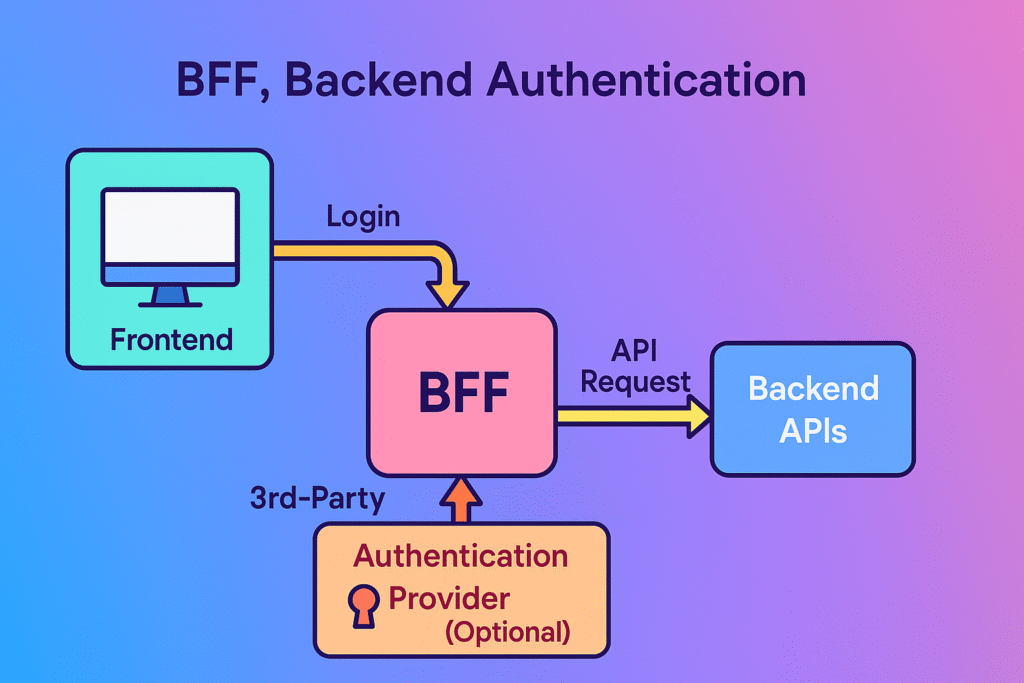
The most common objection to the BFF pattern is that it adds complexity. But let’s challenge that assumption:
The Short-Term vs. Long-Term Complexity Trade-off
Yes, initially you need to set up a backend component. But consider what you’re saving:
- No complex token storage strategies in the frontend
- No need to implement secure token refresh logic in JavaScript
- No worrying about XSS vulnerabilities exposing tokens
- No complex incident response if tokens are compromised
The front-loaded “complexity” of setting up a backend service pays dividends throughout the application lifecycle in terms of reduced security incidents, simplified frontend code, and more reliable user experiences.
Proper Separation of Concerns
Authentication is a core security concern that belongs in your most secure tier (the backend), not in the presentation layer. This separation aligns with fundamental architectural principles.
As noted in a DEV Community article: “While an API Gateway is a single entry point into to the system for all clients, a BFF is only responsible for a single type of client. As mentioned in the text, the most important thing: Avoid storing tokens in the browser. (No tokens in the browser policy).”
This principle—”no tokens in the browser”—is increasingly becoming an industry standard security practice for applications handling sensitive data.
Development Scalability
As your application grows, centralized auth makes it easier to:
- Add new clients (mobile apps, third-party integrations, etc.)
- Implement new security requirements
- Respond to security incidents
- Update authentication flows without modifying clients
According to Microsoft’s Azure Architecture Center documentation, the BFF pattern gives “frontend teams autonomy over their own BFF service, allowing flexibility in language selection, release cadence, workload prioritization, and feature integration.”
A Real-World Case Study: Financial Services Migration
Consider this real-world example: A financial services company previously implemented authentication directly in their Angular SPA, storing tokens in browser localStorage. After a security audit identified this as a significant risk, they migrated to the BFF pattern.
Key benefits they reported:
- Enhanced Security: Removing tokens from the browser eliminated their biggest security concern
- Improved User Experience: Users no longer had to re-authenticate after browser refreshes
- Simplified Frontend Code: The Angular application was simplified by removing token management logic
- Better Monitoring: Centralized authentication made it easier to track and respond to suspicious login attempts
- Regulatory Compliance: The new architecture made it easier to demonstrate compliance with financial regulations
The migration took approximately two development sprints to complete and resulted in a more secure, maintainable system.
The financial services sector is particularly sensitive to authentication security due to regulatory requirements like PSD2 in Europe and various financial privacy regulations in the US. The BFF pattern has become increasingly common in this sector precisely because it improves compliance posture.
When Frontend Auth Might Make Sense
To be fair, there are specific scenarios where frontend authentication could be appropriate:
- Static sites with no backend: If you have a purely static site hosted on a CDN
- Very low-security applications: Internal tools with non-sensitive data
- Prototypes and demos: For quick projects not using real user data
- Public data APIs: If the API only provides public data, the security concerns around tokens might be less critical
But for any production application handling user data, the backend approach provides substantial security benefits.
Even in these cases, as your application grows in importance, transitioning to a backend authentication model provides future-proofing against evolving security threats.
Visualizing the BFF Authentication Flow
Let’s walk through how the BFF pattern works in practice:
- 👤 User clicks “Login” in your SPA
- 🔄 Your SPA redirects to a backend endpoint (e.g.,
/login
) - 🔀 Your backend initiates the OAuth flow, redirecting to the auth server
- 🔐 After authentication, the auth server redirects back with a code
- 🔄 Your backend exchanges this code for tokens and creates a session
- 🍪 The user gets a secure session cookie and returns to your SPA
- 🔄 For API calls, your SPA includes this cookie automatically
- 🔀 Your backend validates the session, attaches the token, and forwards the request
This flow keeps tokens secure while providing a seamless user experience.
The key security improvement here is that sensitive tokens never touch the browser. The only credential that reaches the frontend is a session cookie, which can be secured with HttpOnly, Secure, and SameSite flags to protect against common attack vectors.
How to Implement the BFF Pattern the Right Way
Ready to implement the BFF pattern? Here are some practical tips:
1. Choose the Right Implementation Approach
You have several options:
- Custom Backend Implementation: Build your own if you need maximum control
- Specialized BFF Libraries:
- Spring Addons OIDC (Java)
- OAuth2 Proxy (Go)
- Next-Auth (Next.js)
- Duende BFF (.NET)
- Auth0 SPA SDK with custom backend
- API Gateways with Auth Features:
- Azure API Management
- AWS API Gateway with Lambda authorizers
- Kong with custom plugins
- Zuplo’s API Gateway
- Nginx with auth modules
Each option has its trade-offs in terms of development effort, flexibility, and ongoing maintenance. Your choice should align with your team’s expertise and your application’s specific requirements.
2. Follow These Security Best Practices
For a secure implementation:
- Use short-lived access tokens (15-60 minutes)
- Store refresh tokens securely (encrypted if possible)
- Set HttpOnly, Secure, and SameSite=Strict cookie flags
- Implement CSRF protection for cookie-based authentication
- Log authentication events for security monitoring
- Set up alerts for unusual authentication patterns
- Implement rate limiting on authentication endpoints
- Consider adding additional factors like IP-based validation
A report by Akamai found that 30% of all credential stuffing attacks target financial services applications. Proper implementation of these security practices can dramatically reduce the risk of successful attacks.
3. Make It Developer-Friendly
The BFF pattern should make development easier, not harder:
- Create clear documentation for your frontend developers
- Provide helper libraries or utilities if needed
- Set up proper local development environments that mirror production
- Implement clear error responses that help with debugging
- Create monitoring dashboards for authentication-related metrics
As Microsoft’s Azure Architecture Center documentation notes, the BFF pattern gives “frontend teams autonomy over their own BFF service, allowing flexibility in language selection, release cadence, workload prioritization, and feature integration.”
4. Plan Your API Strategy
With the BFF pattern, all API calls typically flow through your backend. This requires careful planning:
- Design clear API endpoints that mirror your resource server’s endpoints
- Consider performance optimizations like response caching
- Implement proper error handling and status code translation
- Set up monitoring for API performance and availability
- Document the API for frontend developers
This proxying approach also gives you an opportunity to implement additional security measures like request validation, rate limiting, and logging.
Common Questions About the BFF Pattern
Q: Won’t this add latency to my application?
A: The additional latency is minimal—just an extra hop through your backend. The security benefits far outweigh this small performance cost. Plus, your backend can implement caching strategies to offset any latency.
Modern cloud infrastructure makes this additional hop negligible, often adding less than 10ms to request times. Consider that the average human doesn’t perceive delays under 100ms as “slow.”
Q: What about mobile apps?
A: Mobile apps face different security challenges. Modern mobile platforms offer secure storage options like Keychain (iOS) and Keystore (Android). For a fully unified approach, you can still use the BFF pattern with mobile clients.
Many organizations implement a “BFF per client type” approach, with separate BFFs for web, iOS, and Android clients, each optimized for that platform’s specific needs.
Q: How does this work with microservices?
A: The BFF pattern works great with microservices. Your BFF service can authenticate the user and then make authenticated requests to your various microservices.
This approach actually simplifies your microservice architecture by centralizing authentication concerns rather than implementing them in each service.
Q: Does this pattern work with serverless architectures?
A: Absolutely. Your BFF can be implemented as a serverless function that handles authentication and proxies requests. Services like AWS Lambda, Azure Functions, or Google Cloud Functions can effectively implement the BFF pattern with minimal operational overhead.
The Industry Consensus: BFF Is the Safer Choice
This isn’t just one opinion—the security community has reached a clear consensus on this topic:
Auth0 concludes: “If you need a more secure architecture for your SPA, you can apply the Backend for Frontend pattern. This pattern introduces a dedicated backend for token negotiation and management on behalf of the SPA. This way, no tokens will reach the SPA, while the backend will also take charge of proxying the requests to a protected API.”
A DEV Community article on web app security states: “In recent years, it was common to implement OpenID Connect for SPAs in Javascript (React, Angular, Vue…), and this is no longer recommended.” Instead, they advise using the BFF pattern where a server-side component handles tokens.
The IETF OAuth 2.0 working group, Microsoft, and many other security authorities have reached the same conclusion.
The Open Web Application Security Project (OWASP) includes client-side storage of sensitive data in their top ten web application security risks. The BFF pattern directly addresses this risk by keeping sensitive tokens server-side.
Conclusion: Secure by Design
While frontend-managed authentication might seem attractive for its apparent simplicity, it introduces significant security vulnerabilities that aren’t worth the risk for most applications. By keeping sensitive authentication operations on your backend, you’re following security best practices, simplifying token management, and protecting your users’ data more effectively.
The minor additional complexity of implementing backend token exchange is far outweighed by the substantial security benefits and future-proofing it provides.
Remember: In security architecture, convenience should never come at the expense of protecting sensitive credentials. Your authentication flow is the front door to your application—it deserves the strongest locks available.
What’s your next step? If you’re currently using frontend authentication, consider:
- Assessing your current security risk
- Exploring BFF options that fit your technology stack
- Planning a gradual migration, starting with your most security-sensitive features
Your users’ data security is worth the investment. As cyber threats continue to evolve, following industry best practices like the BFF pattern isn’t just good security—it’s good business.
References
- “The Backend for Frontend Pattern (BFF)”, Auth0 Blog, https://auth0.com/blog/the-backend-for-frontend-pattern-bff/
- “OAuth2 Backend for Frontend With Spring Cloud Gateway”, Baeldung, https://www.baeldung.com/spring-cloud-gateway-bff-oauth2
- “Backend For Frontend Authentication Pattern with Auth0 and ASP.NET Core”, Auth0 Blog, https://auth0.com/blog/backend-for-frontend-pattern-with-auth0-and-dotnet/ ↩
- “OAuth 2.0 for Browser-Based Applications”, IETF, https://datatracker.ietf.org/doc/html/draft-ietf-oauth-browser-based-apps
- “OAuth 2.0 Security Best Current Practice”, IETF, https://datatracker.ietf.org/doc/draft-ietf-oauth-security-topics/
- “Web App Security, Understanding the Meaning of the BFF Pattern”, DEV Community, https://dev.to/damikun/web-app-security-understanding-the-meaning-of-the-bff-pattern-i85 ↩ ↩2
- “Backend for Frontend (BFF) Authentication”, Zuplo Blog, https://zuplo.com/blog/2023/09/11/backend-for-frontend-authentication
- “Backend-for-frontend supporting single-page-application implement OIDC/OAuth2 server-side”, GitHub, https://github.com/michaelvl/oidc-oauth2-bff
- “Backends for Frontends pattern”, Azure Architecture Center, https://learn.microsoft.com/en-us/azure/architecture/patterns/backends-for-frontends
- “Application Security Statistics Report”, WhiteHat Security, https://www.whitehatsec.com/blog/application-security-statistics-report/
- “XSS to Account Takeover: Exploiting Token Storage in the Browser”, OWASP Blog, https://owasp.org/blog/2023/token-storage-risks/
- “Data Breach Investigations Report”, Verizon, https://www.verizon.com/business/resources/reports/dbir/
- “E-Commerce Checkout Usability”, Baymard Institute, https://baymard.com/research/checkout-usability
- “State of the Internet Security Report”, Akamai, https://www.akamai.com/internet-security-report
- “OWASP Top Ten Web Application Security Risks”, OWASP, https://owasp.org/www-project-top-ten/
You’re in the right place for those searching for deeper insights and broader perspectives. Explore our curated articles here:
- Facial Recognition with Howdy: Unlock Your Ubuntu 24.0X
- Web 3.0: Dissecting The Hype From Reality
- The Sunlit Path: Can Solar Energy Replace Fossil Fuels?
- The Future of Virtual Reality and the Role of the Metaverse
- The Economic Powerhouse in Your Pocket: How Smartphones Fuel the Economy
- The Curse of Talent: Why Artists Often Grapple with Depression
- Revolutionizing Rhythms Through AI and Web 3.0